- Published on
A Layman's Guide to Leetcode
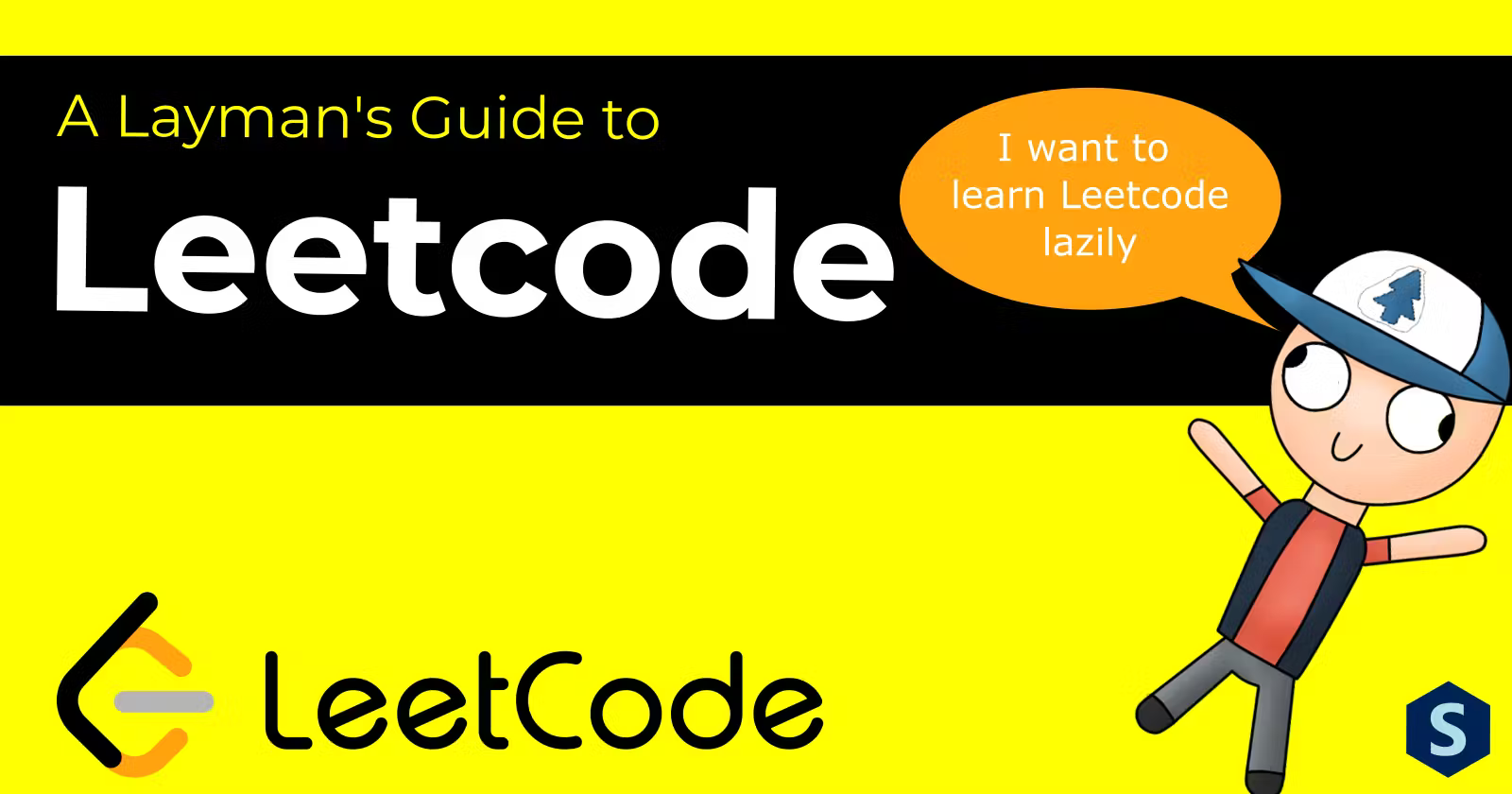
- Authors
- Name
- Hoh Shen Yien
Table of Contents
If you aren't a big fan of algorithms or competitive programming, you'd probably frown at the idea of grinding Leetcode questions. However, given their prevalence as part of the interview process, you might at one point want to prepare yourself for it.
While there are many Leetcode guides out there, this guide is oriented for those who don't plan to put a lot of commitments in Leetcode. Maybe you plan to just put one or two hours per week into it, or even randomly when mood comes, then this is suitable for you.
Of course, you won't become an expert by the end of this guide either. This guide is meant to help you in getting up and running, and even capable of passing some Leetcode interviews, but won't get you into dominating the competitions.
Disclaimer: I won't call myself an expert in these problems (at most decent, I guess), but I can probably solve Medium problems 70% of the time, and some Hard ones with luck.
First Step: Preparation
Programming Language
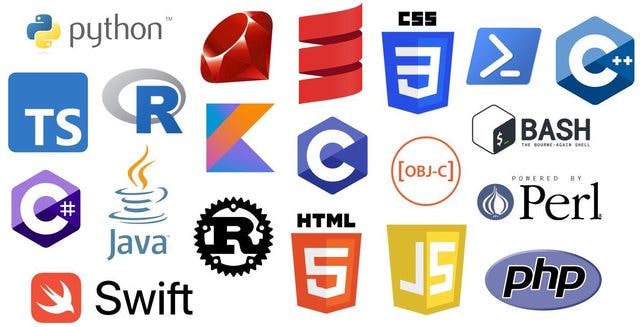
I hope that you do know at least one or two programming languages.
The know here is really basic, you don't need to know any fancy library (actually you might want to know a few data structure libraries) or framework to get started. The absolute minimum requirements to get started with a language are:
- Conditional Statement (if/else)
- Loops (while & for)
- Data Structures (Array / List, Set & Dictionary / HashMap)
- Function
- Basic Object-oriented knowledge
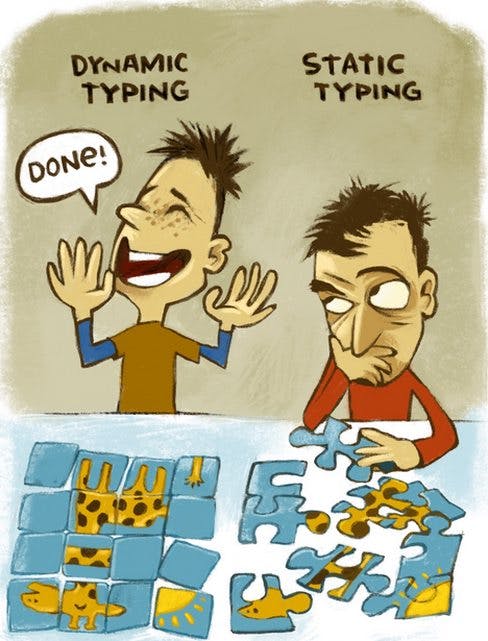
Personally, I recommend dynamically-typed (where you don't have to specify types and integers have no upper limit) and high-level programming languages when solving the problems. You don't have to worry about things like using long or int or be bothered about the return types of recursive functions.
My favorite is Python, but Ruby and JavaScript will do too.
Python has a lot of built-in libraries like heapq and collections that we can use, so I think Python > JavaScript when it comes to problem-solving.
It's nothing wrong to use Java or C in solving Leetcode, it's just that your attention will be diverted to the syntax.
Algorithm Knowledge
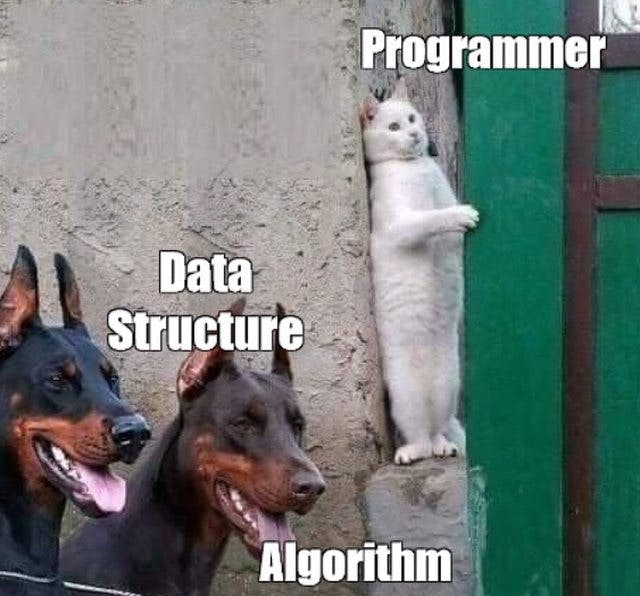
It's not wrong for you to venture into Leetcode without any Data Strcture & Algorithms (DSA) knowledge, but the journey will be painful. (I felt that before)
I would recommend for you to at least know basic concepts (TIme & Space complexity), some algorithms (mainly sorting and searching) and data structures (HashMap, Stack, Queue, Linked List are the most common ones). You can then pick up the techniques (like two pointers and sliding window) along the way.
By the way, the "know" here means that you can at least code it out
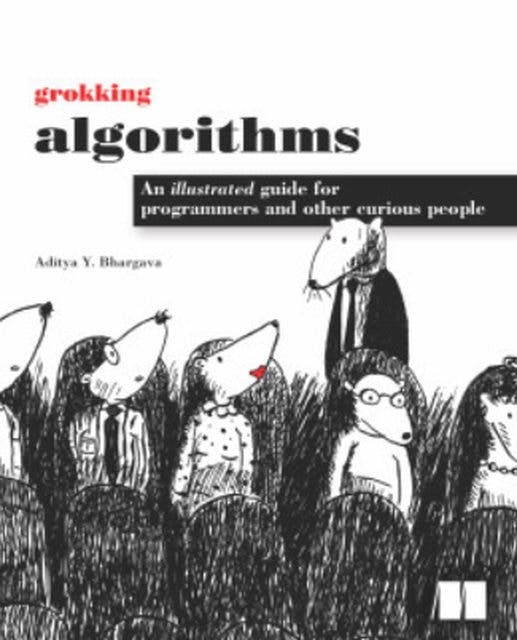
If you're like totally new to these terms, I strongly recommend Grokking Algorithms. Fully illustrated with digestable chunks, very good for you to grasp the basics quickly.
If you have some spare time or prefer video format, I would recommend Algorithms I course (the companion website). There are two parts to the course, but part I will be more than enough to get started.
What about CLRS?
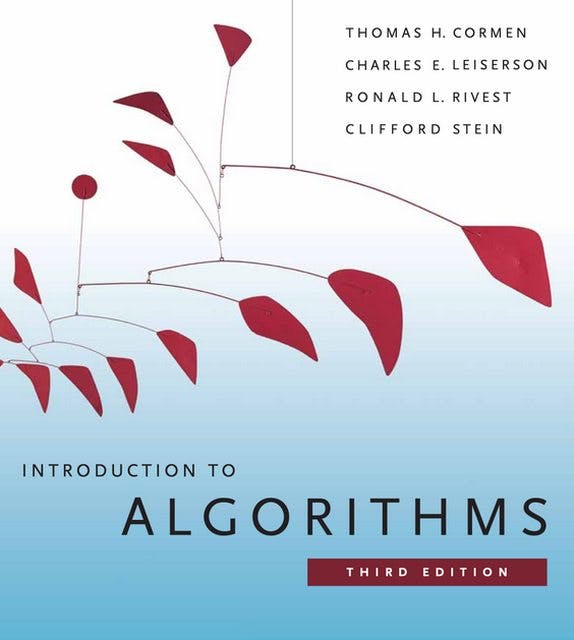
If you've done some searching before, you'd probably come across Introduction to Algorithms (also known as CLRS), or MIT OCW's Introduction to Algorihtms. Don't get me wrong, they're amazing.
After all, we're not going to develop some algorithm libraries or getting our PhD in Computer Science. Our purpose here is to be able to solve some problems right? And those are highly theoretical, definitely not suitable for a layman like you and I. (or maybe just me)
How much to Learn

I believe there are more than thousands of DSAs out there (unproven, this a random figure) but we just need to master a very small subset of those. Trust me, you don't need to know all of them.
Anyways, even if you just know the algorithms that I mentioned earlier, it's all good to get started, certainly more than what I knew back when I started. You'll learn more as you solve more problems along the way.
Into Leetcode
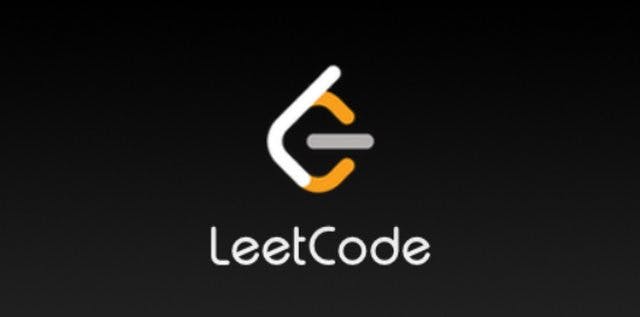
You don't have to practice everyday, but every now and then, you might want to solve a problem or two to get better.
Where to Start — Curated List
So you're ready now, you enter the Leetcode website, and boom, there are thousands of problems! Where should you start? Starting from Problem 1 down the list? or solve all easy problems first?
The key here is to go by topic, more specifically, follow a curated list.
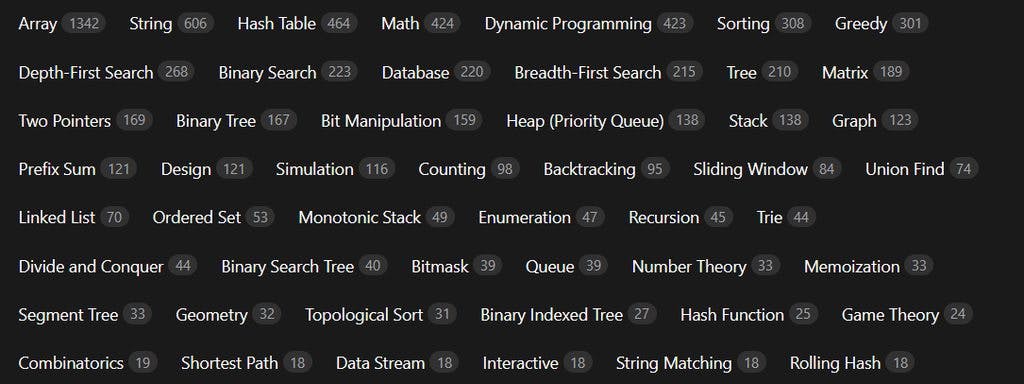
Yes, Leetcode itself has a filter by topics, but even then it's messy. They are unordered and don't build up the difficulty gradually. Trust me, failing to solve most of the problems is a great frustration that stops you from progressing.
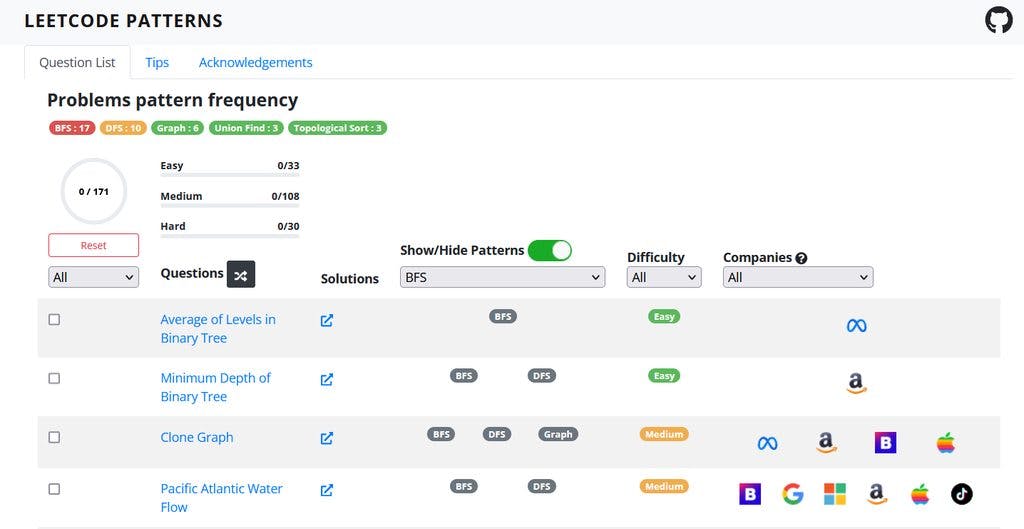
Meanwhile, a curated list by someone not only groups the problem by their topics, they will build up your skills slowly on each topic with increasing difficulty. Just follow along, and you'll find yourself getting better!
So, where's the list? Here are some that I came across in the past
- Blind 75 - Probably the most popular list out there. While the exact topic isn't shown, you will notice that there's a common topic for every few consecutive problems. I highly recommend solving down the list!
- Leetcode Patterns - Another popular list, the best about this list is that they also show how often the problems are asked in popular companies like Google. Nonetheless, you need to remember to filter yourself.
- Leetcode Common Patterns - Quite a decent list too.
All you need to do is to pick one and get started!
Mindset
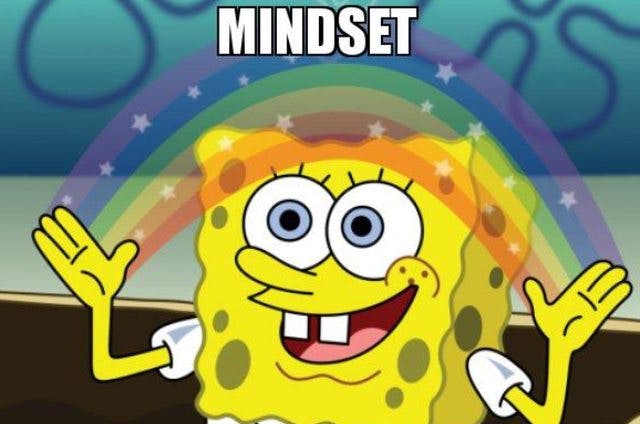
While solving the problem, you have to remember one important thing, we aren't genius. How would we know a technique if we aren't introduced to it?
But before jumping into conclusion that you don't know the technique, check out the related topics first, you might be able to catch a clue or two there.
However, if you get stuck for too long (maybe 30 mins), there's probably still a gap in your knowledge, so stop working, and check the solution.
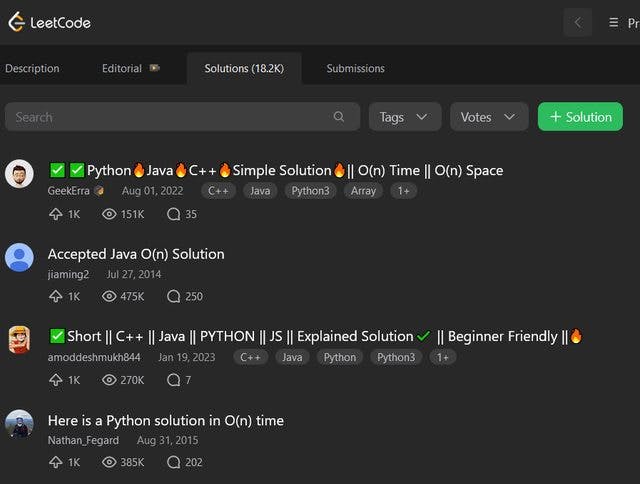
You don't just copy and paste the solution, too! Read thoroughly and understand it. Then, try to answer the problem again without checking back the solution.
PS: There are many solutions for each problem, so if you can't understand one, check for another. You can even search on YouTube for a more comprehensive explanation.
And that's the purpose of using a curated list. You slowly learn about the techniques in each topic, then build up the understanding from the previous problems of the topic.
How Frequent

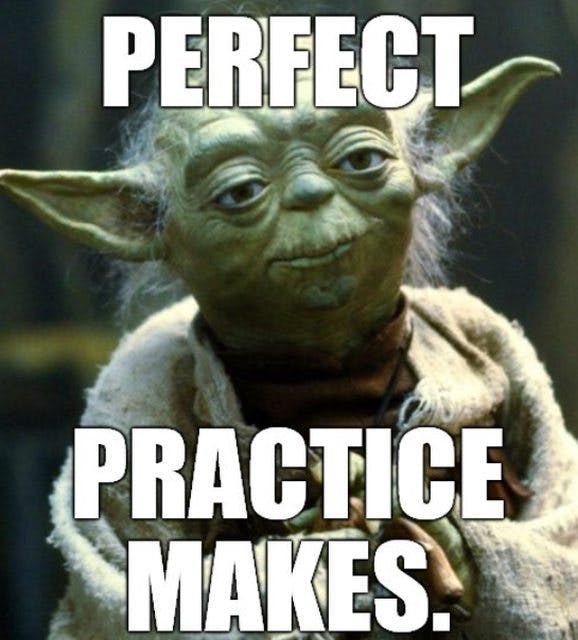
For starters, I'd recommend up to 3-4 problems of the same topic per week. It's even better if you do them on the same day.
Why? You haven't get used to the concepts yet, so it's best to solidify the knowledge in your brain after learning about it from 1 problem, and apply it to other problems.
PS: Of course, the number of problems might need to increase slightly if you find yourself forgetting the techniques after one week.
Nevertheless, after grasping all the topics, you can tone down a little, or even just practice back when you are going to interviews should be sufficient.
However, the frequency totally depends on how fast you want to get good. The faster you wish, you more frequent you should do.
Final Words
I wouldn't even call this guide the best practice, rather, it's just a summary from my past experience with Leetcode.
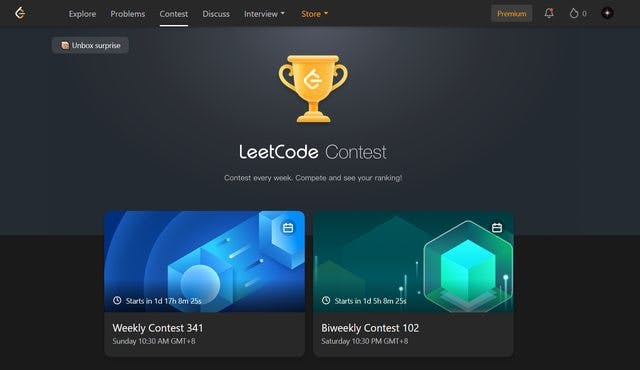
Anyways, you can join some weekly / biweekly contests to see where do you rank (normally, if you can solve 3 out of 4, it's good to go), or even invite some friends to Leetcode together, it can be fun too!
Also, getting the optimal solution might not always be the goal, sometimes the interviewers might be just okay if you provide brute-force solutions. Don't go too harsh on yourself.
Finally, remember that Leetcode is not everything. There are still companies that do technical interviews without Leetcode.